티스토리 뷰
728x90
반응형
애플리케이션을 실행할 때 특정 코드를 실행하고 싶을때 CommandLineRunner 와 ApplicationRunner를 사용한다.
CommnadLineRunner
/**
* Interface used to indicate that a bean should <em>run</em> when it is contained within
* a {@link SpringApplication}. Multiple {@link CommandLineRunner} beans can be defined
* within the same application context and can be ordered using the {@link Ordered}
* interface or {@link Order @Order} annotation.
* <p>
* If you need access to {@link ApplicationArguments} instead of the raw String array
* consider using {@link ApplicationRunner}.
*
* @author Dave Syer
* @since 1.0.0
* @see ApplicationRunner
*/
@FunctionalInterface
public interface CommandLineRunner {
/**
* Callback used to run the bean.
* @param args incoming main method arguments
* @throws Exception on error
*/
void run(String... args) throws Exception;
}
애플리케이션 실행 시 프로그램 아규먼트 (Program Arguments)를 통해 값을 넘겨서 무언가를 실행할 일이 있을 때 사용하면 된다.
jar 파일로 애플리케이션을 실행할때 넘겨서 사용해도 된다.
java -jar ***.jar [value]
사용법
CommandLineRunner 를 구현한 클래스를 @Component로 등록한 후 애플리케이션을 실행하면 된다.
@Component
public class CommandLineRunnerSample implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
Arrays.stream(args)
.forEach(System.out::println);
}
}
### 결과
CommandLineRunner샘플
IDE를 사용하고 있으면 Run/Debug Configuration에서 Program Arguments에 값을 지정해주면 그대로 출력되는것을 확인할 수 있다.
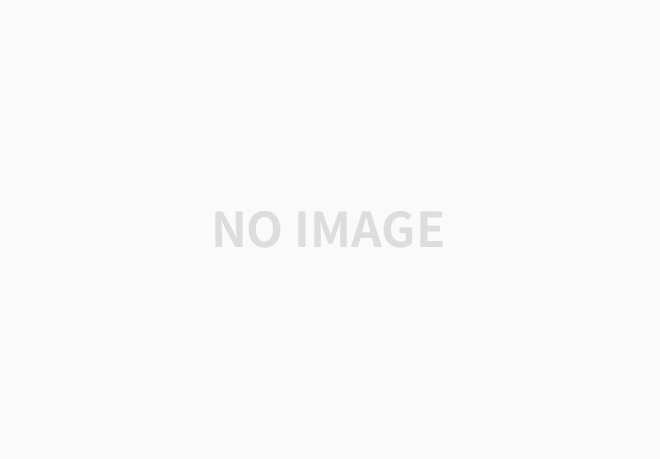
ApplicationRunner
/**
* Interface used to indicate that a bean should <em>run</em> when it is contained within
* a {@link SpringApplication}. Multiple {@link ApplicationRunner} beans can be defined
* within the same application context and can be ordered using the {@link Ordered}
* interface or {@link Order @Order} annotation.
*
* @author Phillip Webb
* @since 1.3.0
* @see CommandLineRunner
*/
@FunctionalInterface
public interface ApplicationRunner {
/**
* Callback used to run the bean.
* @param args incoming application arguments
* @throws Exception on error
*/
void run(ApplicationArguments args) throws Exception;
}
CommandLineRunner 와는 다르게 인자로 ApplicationArguments를 받는다. 애플리케이션 실행 시ApplicationArugments로 입력받은 파라미터를 바인딩 시킨다.
--foo=bar 형식으로 Program Arguments를 넘겨주면 프로퍼티 등에 주입이 가능하다.
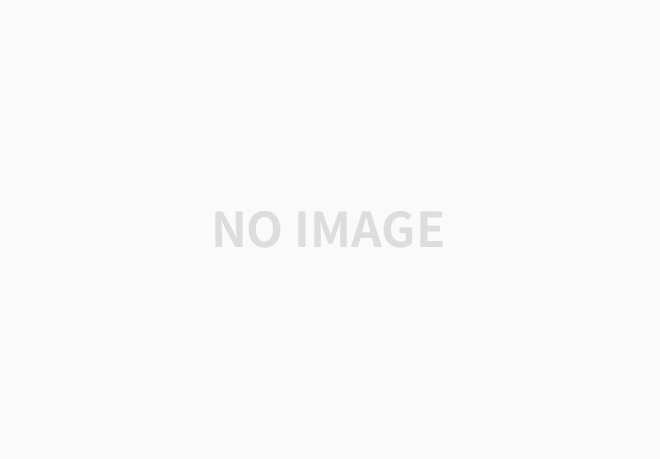
@Component
public class ApplicationRunnerSample implements ApplicationRunner {
@Value("${foo}")
private String foo;
@Override
public void run(ApplicationArguments args) throws Exception {
System.out.println("args.getOptionNames() = " + args.getOptionNames());
System.out.println("args.getOptionValues(\"foo\") = " + args.getOptionValues("foo"));
System.out.println("foo = " + foo);
}
}
### 결과
args.getOptionNames() = [foo]
args.getOptionValues("foo") = [bar]
foo = bar
ApplicationRunner 구현체가 여러개라면?
@Order 애노테이션을 통해서 우선순위를 정할 수 있다. @Order(1), @Order(2) ....
@Order(1)
@Component
public class ApplicationRunnerSample implements ApplicationRunner {
@Override
public void run(ApplicationArguments args) throws Exception {
System.out.printLn("1번");
}
}
@Order(2)
@Component
public class ApplicationRunnerSample2 implements ApplicationRunner {
@Override
public void run(ApplicationArguments args) throws Exception {
System.out.printLn("2번");
}
}
##결과
1번
2번
참고
docs.spring.io/spring-boot/docs/current/reference/htmlsingle/#boot-features-command-line-runner
728x90
반응형
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
TAG
- docker
- Spring Security
- mybatis
- rocky
- svn
- Linux
- config-location
- oracle
- k8s
- LocalDateTime
- mybatis config
- window
- LocalDate
- 오라클
- elasticsearch
- JavaScript
- claude
- 북리뷰
- springboot
- Spring
- Mac
- localtime
- Bash tab
- jQuery
- Kotlin
- input
- Java
- 베리 심플
- maven
- intellij
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
글 보관함
반응형
250x250